Understanding Callbacks in JavaScript
3 min read
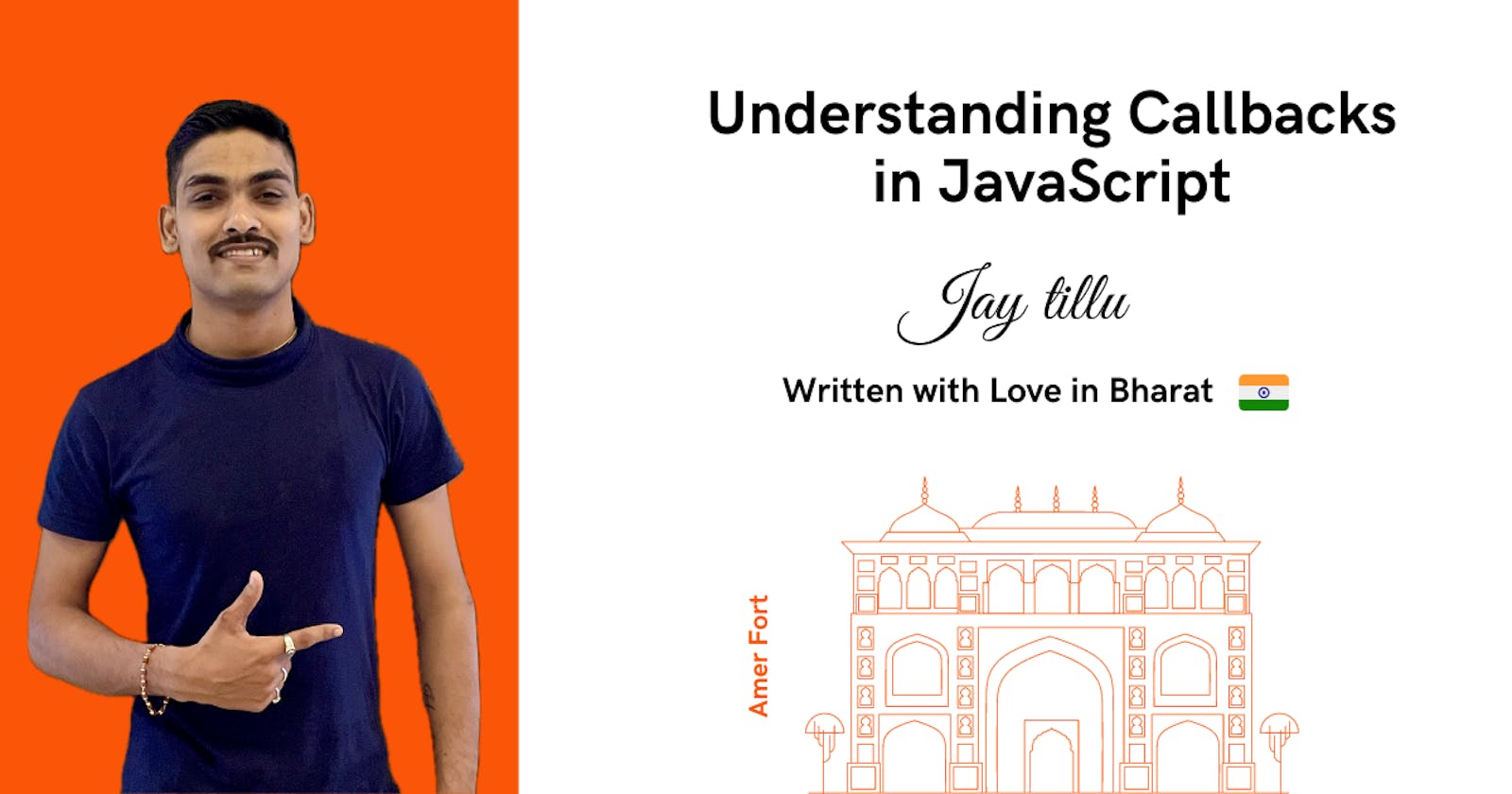
As a language, JavaScript is designed to be asynchronous, allowing developers to create responsive and dynamic web applications. Callbacks play a crucial role in managing asynchronous operations by providing a way to specify what should happen once a particular task is complete. They play a crucial role in JavaScript's event-driven architecture, allowing code to execute when specific events occur.
What is a Callback?
In JavaScript, a callback is simply a function that is passed as an argument to another function. The Callback Function is intended to be executed after the completion of a specific task. This mechanism enables developers to work with asynchronous code, where operations might take some time to finish, like reading a file, making an HTTP request, or handling user input. This mechanism allows for asynchronous programming, where code can continue executing without waiting for the result of a long-running operation.
Example
let h1 = document.querySelector("h1");
function colorChanger(color, duration) {
setTimeout(() => { // setTimeout takes callback function.
h1.style.color = color;
}, duration);
}
colorChanger("yellow", 1000);
Benefits of using Callbacks
Callbacks offer several advantages in JavaScript programming:
Asynchronous Programming: Callbacks enable asynchronous operations, preventing the main thread from being blocked while waiting for long-running tasks. This ensures responsiveness and prevents the user interface from freezing.
Event Handling: Callbacks are essential for handling events in JavaScript. They allow code to execute when specific events occur, such as user interactions, network responses, or timer expirations.
Modular Code: Callbacks promote modularity by encapsulating asynchronous logic into separate functions. This makes code easier to understand, maintain, and reuse.
Callback Hell: A Common Challenge
While callbacks offer flexibility and asynchronous programming capabilities, they can lead to a situation known as "callback hell." This occurs when multiple callbacks are nested within each other, creating a complex and difficult-to-understand code structure.
Avoiding Callback Hell
To avoid callback hell, consider these strategies:
Promises: Promises provide a cleaner and more structured approach to handling asynchronous operations, simplifying code and reducing the risk of callback hell.
Async/Await: Async/await is a newer syntax for asynchronous programming, offering a more readable and concise way to manage asynchronous code.
Modularization: Break down asynchronous logic into separate functions to improve code readability and reduce nesting.
Callback Libraries: Utilize callback libraries like
async
orbluebird
to simplify asynchronous programming and avoid callback hell.
Conclusion
Callbacks are a crucial aspect of JavaScript programming that allows for asynchronous operations and event handling. Although "callback hell" can present difficulties, incorporating techniques such as promises, async/await, and modularization can significantly enhance code readability and maintainability. By understanding callbacks and their nuances, developers can harness their power to create responsive and efficient JavaScript applications.