Value Type and Reference Type in Dart
4 min read
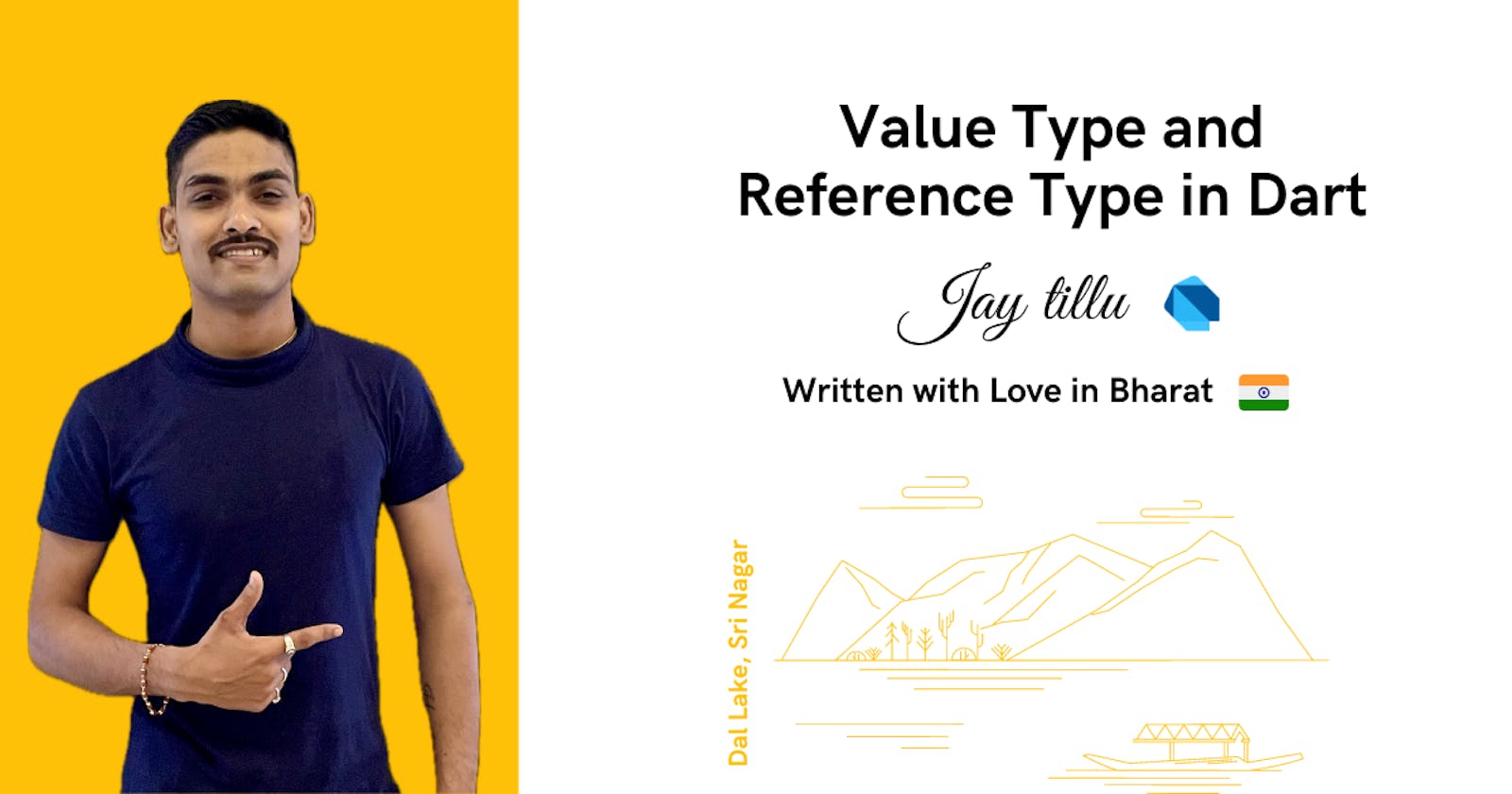
Table of contents
Google's versatile programming language, Dart, is equipped with a strong type system that encompasses two fundamental concepts: value types and reference types. Having a thorough understanding of these concepts is crucial for writing Dart code that is efficient and free of bugs. In this blog post, we will delve into the distinctions between value types and reference types, using illustrative examples to demonstrate their behavior.
Value Types in Dart
In Dart, When you pass primitive data types (such as numbers, strings, or booleans) to a function, a copy of the actual value is passed. Changes made to that variable inside the function do not affect the original variable outside the function. These values are like locked boxes – you can't change what's inside. If you modify the value inside a function, it won't change the original value outside the function because you're working with a separate copy.
In simple terms, Pass by Value is like making a photocopy of a document: changing the copy doesn't affect the original document.
Examples of Value Types in Dart:
Numbers (int, double):
int a = 42;
int b = a; // 'b' gets a copy of the value of 'a'
print(b); // Output: 42
Booleans:
bool isTrue = true;
bool isFalse = isTrue; // 'isFalse' gets a copy of the value of 'isTrue'
print(isFalse); // Output: true
Strings:
String greeting = "Hello";
String copyGreeting = greeting; // 'copyGreeting' gets a copy of the value of 'greeting'
print(copyGreeting); // Output: Hello
Benefits of Value Types in Dart:
Predictable Behavior: Each variable has its own copy of the data, leading to predictable behavior when modifying variables.
Immutability: Value types are often immutable, preventing changes to their values after assignment.
Reference Types in Dart
When you pass a List or Map to a function, a reference to that object is sent to the function. Changes made inside the function affect the original object outside the function.
When you work with non-primitive types like Lists or Maps, they are passed in a way that they share the same underlying data. Imagine it like two people holding a balloon. If one person changes the balloon's color, the other person sees the change because they both have a grip on the same balloon. Similarly, if you modify an object or array inside a function, those changes are reflected outside the function because they both refer to the same object or array in memory.
Examples of Reference Types in Dart:
Lists:
List<int> list1 = [1, 2, 3];
List<int> list2 = list1; // 'list2' refers to the same list as 'list1'
list2[0] = 99;
print(list1); // Output: [99, 2, 3]
Maps:
Map<String, dynamic> map1 = {'key': 'value'};
Map<String, dynamic> map2 = map1; // 'map2' refers to the same map as 'map1'
map2['key'] = 'new value';
print(map1); // Output: {'key': 'new value'}
Benefits of Reference Types:
Efficiency: Reference types facilitate efficient memory usage by avoiding duplication of large amounts of data.
Mutability: Reference types can be mutable, allowing changes to the underlying data.
When to Use Each
Value Types: Use value types when you need independent copies of data, especially for simple, immutable values. This is beneficial in scenarios where changes to one variable should not impact others.
Reference Types: Utilize reference types when you want multiple variables to share and modify the same underlying data. This is useful for complex data structures and situations where memory efficiency is crucial.
Conclusion
Understanding the difference between value types and reference types is crucial for writing clean and efficient Dart code. The decision of when to use each type depends on the specific requirements of your application. By leveraging the strengths of both, you can create robust and maintainable Dart programs.
In conclusion, Dart's type system, with its combination of value types and reference types, provides a flexible foundation for developers. As you continue your Dart journey, a solid understanding of these fundamental concepts will empower you to write more efficient and reliable code. Happy coding!